ANYpay ADK
Overview
ANYpay POS Application Development Kit (ADK) is a library referenced into the Merchant’s POS application. This provides the merchant with the objects and commands necessary to complete an integration in a fastest and simplest way.
With the ANYpay POS ADK, merchant only needs to declare some simple objects and send to the ADK library which prepares the format and content of the information that must be sent to the ANYpay POS to process supported transaction types.
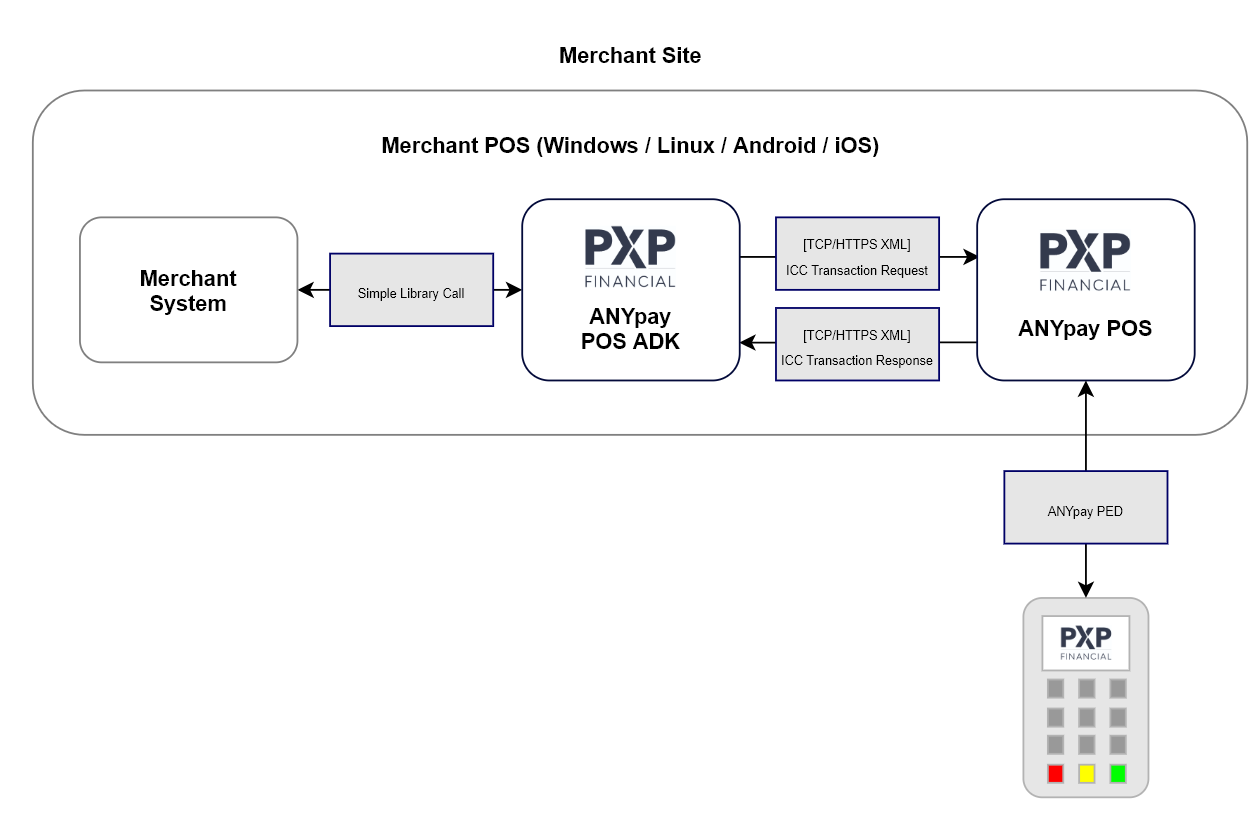
How to reference ANYpay ADK library
- Contact PXP Financial to get an ANYpay ADK package which includes a dll library file, named PXP.ANYpay.POS.ADK.dll.
- Copy the file to a folder in your system.
- Go to Visual Studio to add a reference for your project, and select the dll file.
- Re-build the solution and start to reference to the ANYpay ADK lirbary
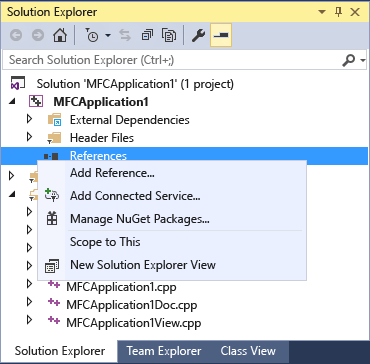
How to start writing the integration code
Find below a sample code written in C# language
//1. COMMUNICATION PROTOCOL
PosClientService _posClientService = new PosTcpClientService(ipAddress: "127.0.0.1", port: 5002);
//2. PED ENDPOINT CONFIGURATION
_posClientService.PedConfig = new PedEndpointAddress
{
EndpointAddressType = PedEndpointAddressType.Serial,
SerialEndpointAddress = new SerialEndpointAddress
{
ComPort = "com9"
}
};
//3. TRANSACTION CONFIGURATION
_posClientService.TransactionConfig = new Credential
{
CustomerCode = "ABF",
Site = "PXP000000001",
Workstation = "001",
Merchant = "1234567890",
Username = "PXPUser",
Password = "Testing",
Culture = "en"
};
//5.1 TRANSACTION REQUEST
var paymentDeviceRequest = new PaymentDeviceRequest
{
Workstation = "001",
AuthorisationType = AuthorisationConnectionType.OnlineAuthorization,
Amount = 10.50M,
Currency = "GBP",
DateTime = DateTime.Now,
TransactionReference = "TransactionReference1",
TransactionType = TransactionType.Sale,
KeyedOnPed = false,
CustomerPresent = false
};
//4. ASYNCHRONOUS/SYNCHRONOUS HANDLING
var paymentDeviceResponse = await _posClientService.ProcessAsync(paymentDeviceRequest);
//5.2 RESPONSE
Console.WriteLine(paymentDeviceResponse.ResponseCode);
Console.WriteLine(paymentDeviceResponse.ResponseMessage);
Console.WriteLine(paymentDeviceResponse.FaultResponseCode);
Console.WriteLine(paymentDeviceResponse.CaptureMethod);
Console.WriteLine(paymentDeviceResponse.AuthorityCode);
Console.WriteLine(paymentDeviceResponse.AuthorityMethod);
Console.WriteLine(paymentDeviceResponse.TokenisedCardNumber);
Console.WriteLine(paymentDeviceResponse.TransactionReference);
Console.WriteLine(paymentDeviceResponse.PEDSerialNumber);
Console.WriteLine(paymentDeviceResponse.Token);
Console.WriteLine(paymentDeviceResponse.ReceiptXML);
1. Communication protocol
This declares the communication details between merchant and ANYpay POS. It must be defined with either TCP, HTTP or HTTPS.
Code samples of Communication protocol can be seen below.
PosClientService _posClientService = new PosTcpClientService()
PosClientService _posClientService = new PosTcpClientService(ipAddress:"123.1.1.1", port:9999)
PosClientService _posClientService = new PosHttpClientService(serviceUrl:"http://localhost:5004")
PosClientService _posClientService = new PosHttpClientService(serviceUrl:"https://localhost:5003")
PosClientService _posClientService = new PosHttpClientService(serviceUrl:"https://localhost:9999",validateServerCetificate:true)
Object | Description | Default |
---|---|---|
PosTcpClientService | ||
ipAddress | The IP address of ANYpay POS | 127.0.0.1 |
port | The port of ANYpay POS | 5002 |
PosHttpClientService | ||
serviceUrl | The http or https url of ANYpay POS. | This field is mandatory |
validateServerCertificate |
The parameter (true/false) to validate the server certificate of ANYpay POS. This parameter is only checked if https url is supplied in serviceUrl. |
false |
2. PED Endpoint configuration
This declares the PED endpoint address details. It must be defined with either TCP or Serial.
Code samples of PED Enpoint address can be seen below.
PedConfig = new PedEndpointAddress
{
EndpointAddressType = PedEndpointAddressType.Tcp,
TcpEndpointAddress = new TcpEndpointAddress
{
HostName = "192.168.204.53",
Port = 16107
}
}
PedConfig = new PedEndpointAddress
{
EndpointAddressType = PedEndpointAddressType.Serial,
SerialEndpointAddress = new SerialEndpointAddress
{
ComPort = "com9"
}
}
Object | Description |
---|---|
PedConfig | |
EndpointAddressType | The endpoint type of the PED |
PedEndpointAddressType | The enum value is either Serial or TCP. |
TcpEndpointAddress | Required if the enpoint type is TCP |
HostName | The IP address/DNS host name of the PED |
Port | The port number of the PED |
SerialEndpointAddress | Required if the enpoint type is Serial |
ComPort | The COM port of the PED |
The pedEndpointConfig can be declared either within the source code or loaded from pre-configured files. See this Configuration files to set up the PED endpoint file
3. Transaction configuration
This declares the workstation details.
A code sample of Transaction configuration can be seen below.
TransactionConfig = new Credential
{
CustomerCode = "ABF",
Site = "PXP000000001",
Workstation = "001",
Merchant = "1234567890",
Username = "PXPUser",
Password = "Testing",
Culture = "en"
}
The transactionConfig can be declared either within the source code or loaded from pre-configured files. See this Configuration files to set up the transactionConfig file.
Object | Description |
---|---|
CustomerCode | This is the customer code, a unique customer ID allocated to a customer when the service is set up |
Site | This is the site identifier, a unique site ID allocated to a customer when the service is set up. A site can be a till point or a server |
Culture | This is the language used for messages displayed on the PIN Entry Device |
Workstation | This is the workstation identifier. The workstation can be a till point or server |
MerchantId | This is the merchant identification number (MID) assigned to the merchant by the merchant's acquirer |
Username | This is a username allocated to a service user when the service is set up; it refers to a service account not a person |
Password | This is a password allocated to a service user when the service is set up; it refers to a service account not a person |
IpAddress | This is the source of the transaction (a till point or server) |
4. Asynchronous or Synchronous process
The transaction can be processed with either sync/async, code samples can be seen below.
var Response = await _posClientService.ProcessAsync(Request);
var Response = _posClientService.ProcessAsync(Request).Result;
5. Transaction requests/responses
There are some code samples for each request type that ANYpay ADK currently supports, see below for more details.
Request Type | Description |
---|---|
Payment Device Request | This request supports customer present transaction which involves PED interaction. |
Token Request | This request supports transaction processed with PXP token/tokenized card number. |
Topup Request | This request supports incremental authorizsation transaction processed with PXP tokenized card number. |
Capture Request | This request supports capture authorizsation transaction processed with PXP tokenized card number. |
Reversal Request | This request supports reversal transaction processed with PXP tokenized card number. |
Get Transaction Status Request | This request supports to query a status of a transaction using the transaction reference. |
Account Verification Request | This request supports to check a payment card valid or not. |
Get Token From Payment Device Request | This request supports to get a PXP tokenized card number. |
PED Status Request | This request supports to query the status of the PED. |
POS Status Request | This request supports to query the status of the POS. |
Status Packet | This request supports the status during transaction process. |
Updated about 4 years ago